
The Hidden Power of crypto-js npm: Revealed!
In today’s digital landscape, security is paramount. As web developers, we’re constantly seeking robust tools to protect sensitive data and ensure the integrity of our applications. Enter crypto-js npm, a powerful JavaScript library that brings advanced cryptographic capabilities to your projects. Whether you’re building a secure authentication system or implementing end-to-end encryption, crypto-js npm offers a versatile toolkit to meet your cryptographic needs.
In this comprehensive guide, we’ll dive deep into the world of crypto-js npm, exploring its features, use cases, and best practices. We’ll walk you through everything from basic setup to advanced implementations, helping you harness the full potential of this essential cryptography library.

Understanding Crypto-js NPM
What is crypto-js npm?
Crypto-js npm is a JavaScript library that provides a collection of cryptographic functions implemented purely in JavaScript. It’s designed to work in both Node.js environments and modern web browsers, making it a versatile choice for developers working on various platforms.
The library offers a wide range of cryptographic algorithms, including:
- Hashing functions (MD5, SHA-1, SHA-256, etc.)
- Symmetric encryption (AES, DES, Triple DES, etc.)
- HMAC (Hash-based Message Authentication Code)
- PBKDF2 (Password-Based Key Derivation Function 2)
These functions allow developers to perform essential cryptographic operations without the need for complex mathematical implementations or native bindings to system-level cryptographic libraries.
History and development of crypto-js
Crypto-js has been around for quite some time, with its roots tracing back to the early days of JavaScript cryptography. The project was initially created by Jeff Mott and has since been maintained by a dedicated community of developers.
Over the years, crypto-js has evolved to keep pace with modern cryptographic standards and best practices. The npm package, which we’ll be focusing on in this guide, has made it even easier for developers to integrate crypto-js into their projects.
Features and capabilities of crypto-js npm
Let’s take a closer look at some of the key features that make crypto-js npm a go-to choice for JavaScript cryptography:
- Pure JavaScript implementation: Crypto-js is written entirely in JavaScript, ensuring compatibility across different platforms and environments.
- Comprehensive algorithm support: The library includes a wide range of cryptographic algorithms, from basic hashing functions to advanced encryption schemes.
- Flexible encoding options: Crypto-js supports various data encodings, including UTF-8, Base64, and Hex, making it easy to work with different data formats.
- Cross-platform compatibility: Whether you’re developing for Node.js or the browser, crypto-js npm has got you covered.
- Active community and maintenance: With regular updates and a responsive community, you can rely on crypto-js npm to stay current with the latest security standards.
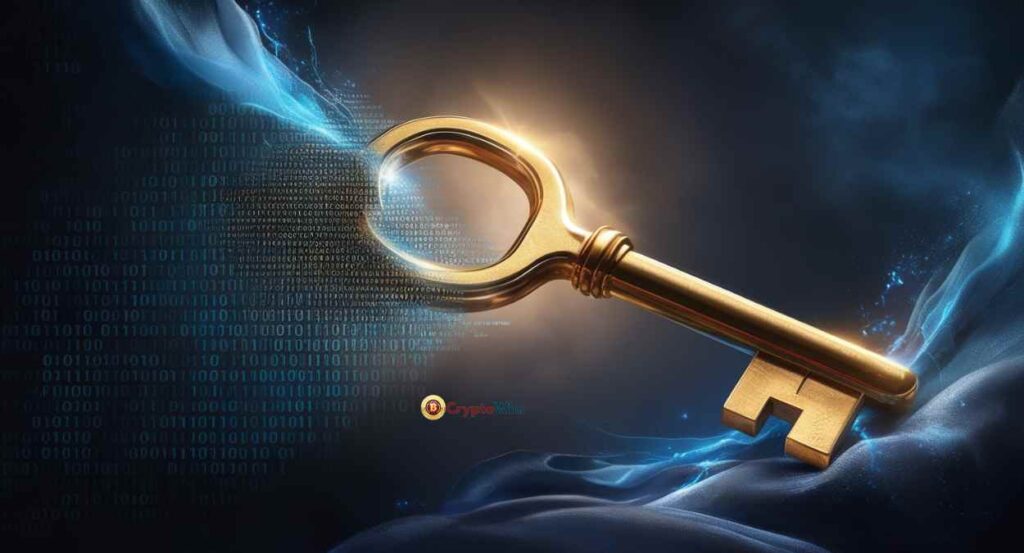
Getting Started with Crypto-js NPM
Now that we’ve covered the basics, let’s dive into how you can start using crypto-js npm in your projects.
Installation process
Installing crypto-js npm is straightforward. Open your terminal and run the following command in your project directory:
npm install crypto-js
This will download and install the latest version of crypto-js npm and add it to your project’s dependencies.
Basic setup and configuration
Once installed, you can import crypto-js into your JavaScript files. Here’s a basic example of how to import and use the library:
const CryptoJS = require('crypto-js');
// Example: Hashing a string using SHA-256
const hash = CryptoJS.SHA256('Hello, World!');
console.log(hash.toString());
In this simple example, we’re using the SHA-256 hashing function to create a hash of the string “Hello, World!”. The toString()
method converts the hash object to a hexadecimal string representation.
Importing crypto-js into your project
For more specific use cases, you can import only the functions you need, which can help reduce your bundle size in browser environments:
const SHA256 = require('crypto-js/sha256');
const AES = require('crypto-js/aes');
// Use SHA256 and AES as needed
This approach allows you to cherry-pick the specific algorithms you need, potentially improving performance and reducing your application’s footprint.
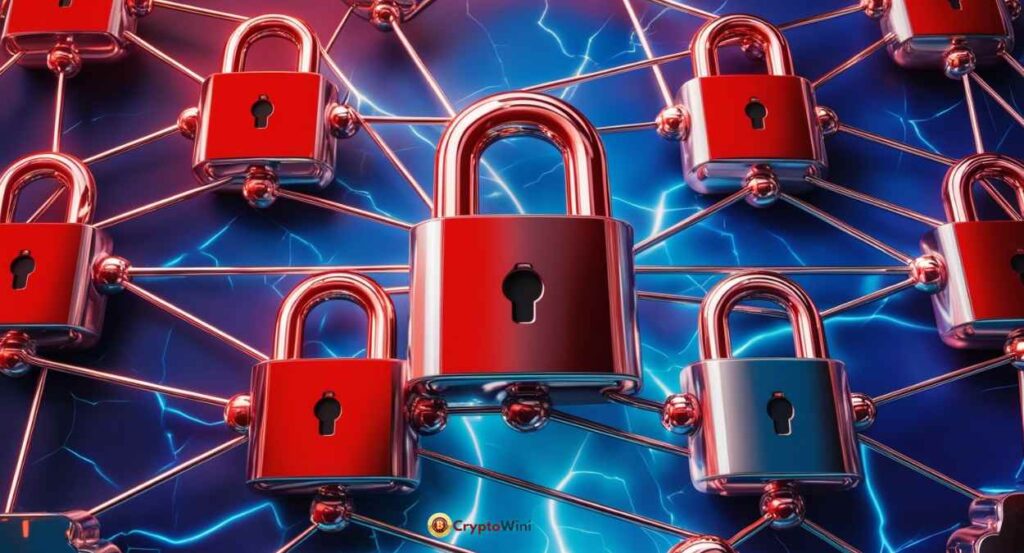
Core Functionalities of Crypto-js NPM
Now that we’ve covered the basics, let’s explore the core functionalities of crypto-js npm in more detail.
Hashing Algorithms
Hashing is a fundamental operation in cryptography, used for creating fixed-size representations of data. Crypto-js npm supports several popular hashing algorithms:
MD5
While MD5 is no longer considered cryptographically secure, it’s still used in some legacy systems and for non-security-critical applications like checksums.
const MD5 = require('crypto-js/md5');
const hash = MD5('Your message here');
console.log(hash.toString());
SHA-1
Like MD5, SHA-1 is no longer recommended for security-critical applications but remains in use for certain purposes.
const SHA1 = require('crypto-js/sha1');
const hash = SHA1('Your message here');
console.log(hash.toString());
SHA-2 (SHA-256, SHA-512)
SHA-2 algorithms, particularly SHA-256 and SHA-512, are widely used and considered secure for most applications.
const SHA256 = require('crypto-js/sha256');
const SHA512 = require('crypto-js/sha512');
const hash256 = SHA256('Your message here');
const hash512 = SHA512('Your message here');
console.log(hash256.toString());
console.log(hash512.toString());
Encryption and Decryption
Crypto-js npm provides several encryption algorithms for securing data. Let’s look at some of the most commonly used ones:
AES Encryption
Advanced Encryption Standard (AES) is a symmetric encryption algorithm widely used for securing sensitive data.
const AES = require('crypto-js/aes');
const enc = require('crypto-js/enc-utf8');
const secretKey = 'my-secret-key';
const message = 'This is a secret message';
// Encryption
const encrypted = AES.encrypt(message, secretKey).toString();
console.log('Encrypted:', encrypted);
// Decryption
const decrypted = AES.decrypt(encrypted, secretKey);
console.log('Decrypted:', decrypted.toString(enc));
DES and Triple DES
Data Encryption Standard (DES) and Triple DES are older encryption algorithms. While they’re not recommended for new applications due to security concerns, they may be needed for compatibility with legacy systems.
const DES = require('crypto-js/tripledes');
const enc = require('crypto-js/enc-utf8');
const secretKey = 'my-secret-key';
const message = 'This is a secret message';
// Encryption
const encrypted = DES.encrypt(message, secretKey).toString();
console.log('Encrypted:', encrypted);
// Decryption
const decrypted = DES.decrypt(encrypted, secretKey);
console.log('Decrypted:', decrypted.toString(enc));
Rabbit
Rabbit is a high-speed stream cipher that can be useful for applications requiring fast encryption of large amounts of data.
const Rabbit = require('crypto-js/rabbit');
const enc = require('crypto-js/enc-utf8');
const secretKey = 'my-secret-key';
const message = 'This is a secret message';
// Encryption
const encrypted = Rabbit.encrypt(message, secretKey).toString();
console.log('Encrypted:', encrypted);
// Decryption
const decrypted = Rabbit.decrypt(encrypted, secretKey);
console.log('Decrypted:', decrypted.toString(enc));
HMAC (Hash-based Message Authentication Code)
HMAC is used to verify both the integrity and authenticity of a message. It combines a cryptographic hash function with a secret key.
const HmacSHA256 = require('crypto-js/hmac-sha256');
const message = 'This is a message';
const secretKey = 'my-secret-key';
const hmac = HmacSHA256(message, secretKey);
console.log('HMAC:', hmac.toString());
PBKDF2 (Password-Based Key Derivation Function 2)
PBKDF2 is used to derive a secure key from a password or passphrase. It’s particularly useful for password hashing and key generation.
const PBKDF2 = require('crypto-js/pbkdf2');
const password = 'user-password';
const salt = 'random-salt';
const iterations = 10000;
const keySize = 256 / 32; // 256 bits
const key = PBKDF2(password, salt, { keySize: keySize, iterations: iterations });
console.log('Derived Key:', key.toString());
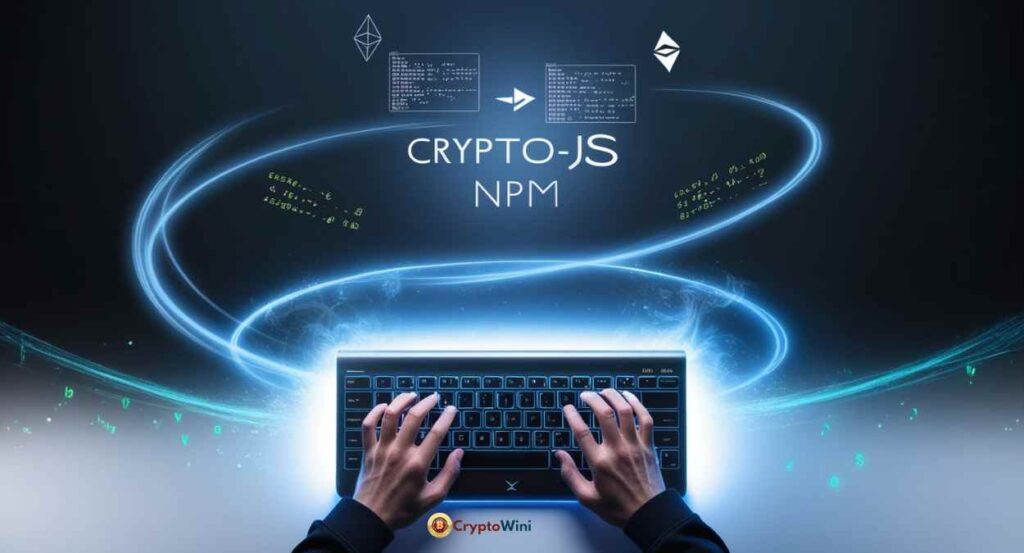
Advanced Usage of Crypto-js NPM
As you become more comfortable with the basics of crypto-js npm, you can explore more advanced use cases and optimizations.
Working with different encodings
Crypto-js npm supports various encodings, allowing you to work with data in different formats:
const CryptoJS = require('crypto-js');
const message = 'Hello, World!';
// Convert to different encodings
const base64 = CryptoJS.enc.Base64.stringify(CryptoJS.enc.Utf8.parse(message));
const hex = CryptoJS.enc.Hex.stringify(CryptoJS.enc.Utf8.parse(message));
console.log('Base64:', base64);
console.log('Hex:', hex);
// Convert back to UTF-8
const fromBase64 = CryptoJS.enc.Base64.parse(base64).toString(CryptoJS.enc.Utf8);
const fromHex = CryptoJS.enc.Hex.parse(hex).toString(CryptoJS.enc.Utf8);
console.log('From Base64:', fromBase64);
console.log('From Hex:', fromHex);
Implementing custom algorithms
While crypto-js npm provides a wide range of built-in algorithms, you can also implement custom algorithms if needed:
const CryptoJS = require('crypto-js');
// Custom algorithm example: XOR cipher
CryptoJS.lib.Cipher.extend({
_doReset: function () {
this._xorKey = this.cfg.key;
},
encryptBlock: function (words, offset) {
for (let i = 0; i < 4; i++) {
words[offset + i] ^= this._xorKey[i];
}
},
decryptBlock: function (words, offset) {
this.encryptBlock(words, offset);
}
});
// Use the custom algorithm
const key = CryptoJS.enc.Utf8.parse('my-secret-key');
const message = 'This is a secret message';
const encrypted = CryptoJS.lib.Cipher.create({ key: key }).finalize(message);
console.log('Encrypted:', encrypted.toString());
const decrypted = CryptoJS.lib.Cipher.create({ key: key }).finalize(encrypted);
console.log('Decrypted:', decrypted.toString(CryptoJS.enc.Utf8));
Performance optimization techniques
When working with large amounts of data or in performance-critical applications, consider these optimization techniques:
- Use typed arrays: For large data sets, using typed arrays can improve performance:
const CryptoJS = require('crypto-js');
const data = new Uint8Array(1000000); // Large data set
const wordArray = CryptoJS.lib.WordArray.create(data);
const hash = CryptoJS.SHA256(wordArray);
console.log('Hash:', hash.toString());
- Progressive hashing: For streaming data or large files, use progressive hashing:
const CryptoJS = require('crypto-js');
const sha256 = CryptoJS.algo.SHA256.create();
// Simulate streaming data
for (let i = 0; i < 10; i++) {
sha256.update('chunk of data');
}
const hash = sha256.finalize();
console.log('Hash:', hash.toString());
Error handling and security best practices
When implementing cryptography, proper error handling and following security best practices are crucial:
- Use try-catch blocks: Always wrap cryptographic operations in try-catch blocks to handle potential errors gracefully:
const CryptoJS = require('crypto-js');
try {
const encrypted = CryptoJS.AES.encrypt('message', 'key').toString();
console.log('Encrypted:', encrypted);
} catch (error) {
console.error('Encryption failed:', error.message);
}
- Secure key management: Never hardcode keys in your source code. Use environment variables or secure key management systems:
const CryptoJS = require('crypto-js');
const secretKey = process.env.SECRET_KEY;
if (!secretKey) {
throw new Error('Secret key not found in environment variables');
}
const encrypted = CryptoJS.AES.encrypt('message', secretKey).toString();
console.log('Encrypted:', encrypted);
- Use constant-time comparison: When comparing hashes or MACs, use constant-time comparison to prevent timing attacks:
const CryptoJS = require('crypto-js');
function constantTimeEqual(a, b) {
if (a.length !== b.length) {
return false;
}
let result = 0;
for (let i = 0; i < a.length; i++) {
result |= a.charCodeAt(i) ^ b.charCodeAt(i);
}
return result === 0;
}
const hmac1 = CryptoJS.HmacSHA256('message', 'key').toString();
const hmac2 = CryptoJS.HmacSHA256('message', 'key').toString();
console.log('HMACs equal:', constantTimeEqual(hmac1, hmac2));
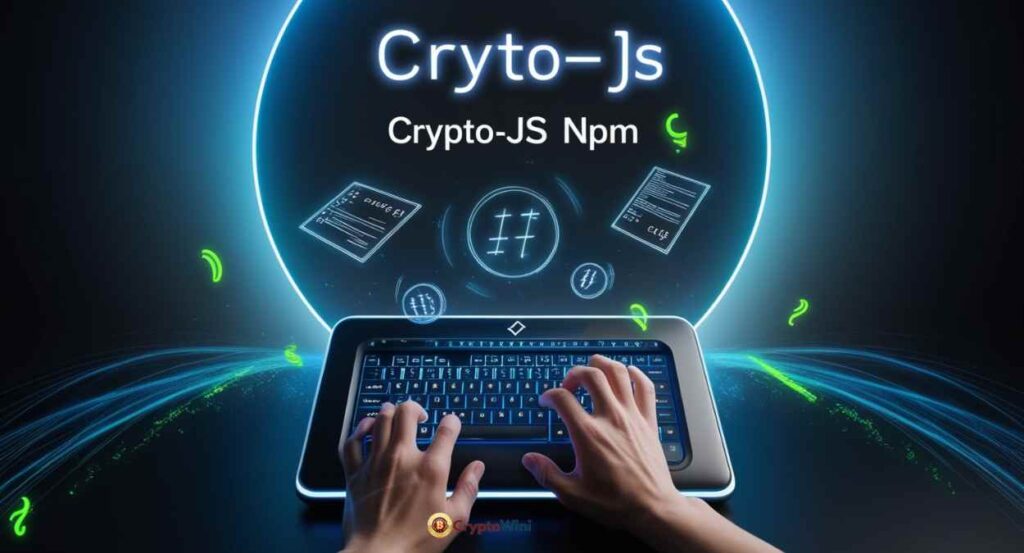
Crypto-js NPM in Real-World Applications
Now that we’ve covered the core functionalities and advanced usage of crypto-js npm, let’s explore how it can be applied in real-world scenarios.
Securing user authentication
Crypto-js npm can be used to enhance the security of user authentication systems. Here’s an example of how you might hash and verify passwords:
const CryptoJS = require('crypto-js');
function hashPassword(password, salt) {
return CryptoJS.PBKDF2(password, salt, {
keySize: 256 / 32,
iterations: 10000
}).toString();
}
function verifyPassword(inputPassword, storedHash, salt) {
const inputHash = hashPassword(inputPassword, salt);
return inputHash === storedHash;
}
// Usage
const password = 'user-password';
const salt = CryptoJS.lib.WordArray.random(128 / 8).toString();
const hashedPassword = hashPassword(password, salt);
console.log('Hashed Password:', hashedPassword);
const isValid = verifyPassword('user-password', hashedPassword, salt);
console.log('Password Valid:', isValid);
Protecting sensitive data in transit
When sending sensitive data over the network, you can use crypto-js npm to encrypt the data before transmission:
const CryptoJS = require('crypto-js');
function encryptData(data, key) {
return CryptoJS.AES.encrypt(JSON.stringify(data), key).toString();
}
function decryptData(encryptedData, key) {
const bytes = CryptoJS.AES.decrypt(encryptedData, key);
return JSON.parse(bytes.toString(CryptoJS.enc.Utf8));
}
// Usage
const sensitiveData = { username: 'john_doe', creditCard: '1234-5678-9012-3456' };
const secretKey = 'my-secret-key';
const encrypted = encryptData(sensitiveData, secretKey);
console.log('Encrypted:', encrypted);
const decrypted = decryptData(encrypted, secretKey);
console.log('Decrypted:', decrypted);
Implementing digital signatures
Digital signatures can be used to verify the authenticity and integrity of messages. Here’s a simple implementation using crypto-js npm:
function signMessage(message, privateKey) {
return CryptoJS.HmacSHA256(message, privateKey).toString();
}
function verifySignature(message, signature, publicKey) {
const computedSignature = CryptoJS.HmacSHA256(message, publicKey).toString();
return computedSignature === signature;
}
// Usage
const message = 'This is a confidential message';
const privateKey = 'private-key';
const publicKey = 'public-key';
const signature = signMessage(message, privateKey);
console.log('Signature:', signature);
const isValid = verifySignature(message, signature, publicKey);
console.log('Signature Valid:', isValid);
Creating secure communication channels
Crypto-js npm can be used to implement secure communication channels between clients and servers. Here’s a simplified example of how you might set up encrypted communication:
const CryptoJS = require('crypto-js');
class SecureChannel {
constructor(sharedSecret) {
this.sharedSecret = sharedSecret;
}
encrypt(message) {
return CryptoJS.AES.encrypt(message, this.sharedSecret).toString();
}
decrypt(encryptedMessage) {
const bytes = CryptoJS.AES.decrypt(encryptedMessage, this.sharedSecret);
return bytes.toString(CryptoJS.enc.Utf8);
}
}
// Usage
const channel = new SecureChannel('shared-secret-key');
const message = 'Top secret information';
const encrypted = channel.encrypt(message);
console.log('Encrypted:', encrypted);
const decrypted = channel.decrypt(encrypted);
console.log('Decrypted:', decrypted);
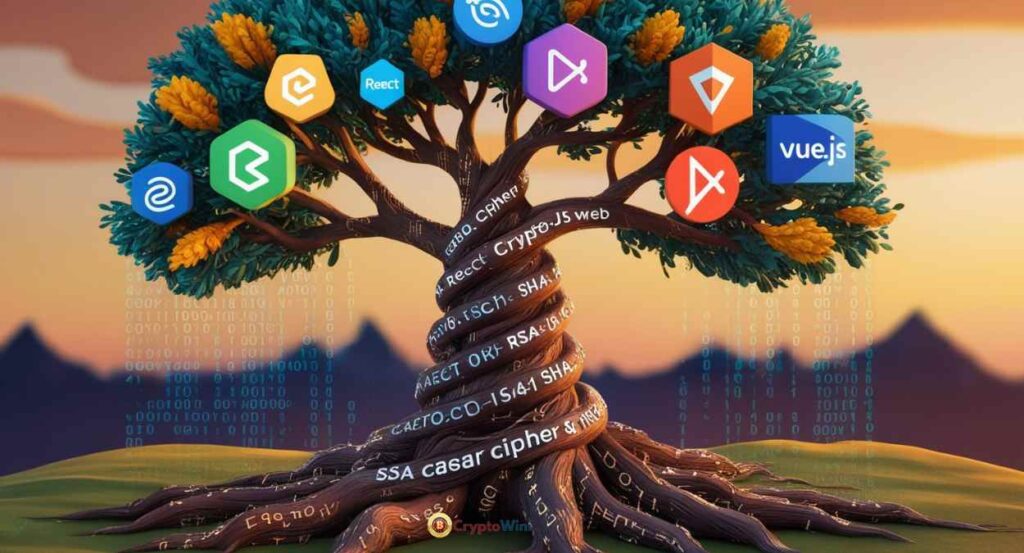
Comparing Crypto-js NPM to Other Cryptography Libraries
While crypto-js npm is a powerful and versatile library, it’s worth comparing it to other options available in the JavaScript ecosystem.
Crypto-js vs. Node.js built-in crypto module
Node.js comes with a built-in crypto
module that provides cryptographic functionality. Here’s a comparison:
Feature | Crypto-js NPM | Node.js crypto |
---|---|---|
Platform | Browser & Node.js | Node.js only |
Implementation | Pure JavaScript | C/C++ bindings |
Performance | Good | Excellent |
Ease of use | High | Moderate |
Algorithm support | Extensive | Extensive |
Example using Node.js crypto:
const crypto = require('crypto');
const hash = crypto.createHash('sha256').update('Hello, World!').digest('hex');
console.log('Hash:', hash);
Crypto-js vs. Web Crypto API
The Web Crypto API is a browser-based cryptography API. Here’s how it compares to crypto-js npm:
Feature | Crypto-js NPM | Web Crypto API |
---|---|---|
Platform | Browser & Node.js | Browser only |
Implementation | Pure JavaScript | Native |
Performance | Good | Excellent |
Ease of use | High | Moderate |
Algorithm support | Extensive | Limited |
Example using Web Crypto API:
async function sha256(message) {
const msgBuffer = new TextEncoder().encode(message);
const hashBuffer = await crypto.subtle.digest('SHA-256', msgBuffer);
const hashArray = Array.from(new Uint8Array(hashBuffer));
return hashArray.map(b => b.toString(16).padStart(2, '0')).join('');
}
sha256('Hello, World!').then(hash => console.log('Hash:', hash));
Advantages and limitations of crypto-js npm
Advantages:
- Cross-platform compatibility
- Extensive algorithm support
- Easy to use and integrate
- Active community and maintenance
Limitations:
- Performance may be slower than native implementations
- Larger bundle size compared to more focused libraries
- Not suitable for high-security applications requiring hardware-level security

Best Practices and Security Considerations
When working with cryptography, it’s crucial to follow best practices to ensure the security of your applications.
Choosing the right algorithms for your needs
- Use AES for symmetric encryption
- Prefer SHA-256 or SHA-3 for hashing
- Use PBKDF2 or bcrypt for password hashing
- Implement HMAC for message authentication
Key management and storage
- Never hardcode keys in your source code
- Use environment variables or secure key management systems
- Rotate keys regularly
- Use different keys for different purposes
Avoiding common cryptographic mistakes
- Don’t create your own cryptographic algorithms
- Use secure random number generators for key generation
- Implement proper error handling
- Be aware of side-channel attacks
Keeping crypto-js npm up to date
- Regularly update to the latest version of crypto-js npm
- Monitor for security advisories related to the library
- Test your application thoroughly after updates

Future of Crypto-js NPM
As the field of cryptography evolves, so too does crypto-js npm. Here’s what we might expect in the future:
Upcoming features and improvements
- Support for post-quantum cryptography algorithms
- Enhanced performance optimizations
- Expanded TypeScript support
Community contributions and support
The open-source nature of crypto-js npm means that community contributions play a vital role in its development. Developers can contribute by:
- Reporting bugs and security issues
- Submitting pull requests for new features or improvements
- Helping to improve documentation
Adapting to evolving cryptographic standards
As new cryptographic standards emerge, crypto-js npm will likely adapt to include:
- Support for new hash functions (e.g., SHA-3 variants)
- Implementation of newer encryption algorithms
- Compliance with updated security recommendations
Conclusion
Crypto-js npm stands as a versatile and powerful tool in the JavaScript developer’s cryptographic arsenal. Its wide range of supported algorithms, ease of use, and cross-platform compatibility make it an excellent choice for many cryptographic needs.
Throughout this guide, we’ve explored the core functionalities of crypto-js npm, from basic hashing and encryption to more advanced use cases. We’ve seen how it can be applied in real-world scenarios, such as securing user authentication and protecting sensitive data in transit.
While crypto-js npm has its limitations, particularly in terms of performance compared to native implementations, its flexibility and extensive feature set make it a valuable library for many applications. As with any cryptographic tool, it’s crucial to stay informed about best practices and keep your dependencies up to date to ensure the security of your applications.
As the cryptographic landscape continues to evolve, crypto-js npm is likely to adapt and grow, maintaining its position as a go-to cryptography library for JavaScript developers. Whether you’re building a simple secure communication channel or implementing complex cryptographic protocols, crypto-js npm provides the tools you need to add robust security to your JavaScript applications.
FAQs
Q. What is the main purpose of crypto-js npm?
A. Crypto-js npm is a JavaScript library that provides a wide range of cryptographic functions, including hashing, encryption, and key derivation. Its main purpose is to enable developers to implement cryptographic operations in both browser and Node.js environments using pure JavaScript.
Q. How does crypto-js npm compare to native cryptography implementations?
A. While crypto-js npm offers excellent cross-platform compatibility and ease of use, it may not match the performance of native cryptography implementations like the Node.js crypto module or Web Crypto API. However, it provides a wider range of algorithms and is often more flexible for various use cases.
Q. Is crypto-js npm suitable for high-security applications?
A. Crypto-js npm is generally secure for many applications, but for high-security scenarios requiring hardware-level security or compliance with specific standards, it may be more appropriate to use platform-specific cryptographic libraries or specialized security hardware.
Q. How often should I update crypto-js npm in my projects?
A. It’s recommended to keep crypto-js npm up to date with the latest stable version. Regularly check for updates and security advisories, and plan to update at least quarterly or whenever a new version with security fixes is released.
Q. Can crypto-js npm be used for password hashing?
A. Yes, crypto-js npm can be used for password hashing. It provides functions like PBKDF2 which are suitable for password hashing. However, for production systems, consider using specialized password hashing libraries like bcrypt or Argon2.
Q. How does crypto-js npm handle key management?
A. Crypto-js npm provides the cryptographic functions, but key management is the responsibility of the developer. It’s crucial to implement secure key generation, storage, and rotation practices in your application when using crypto-js npm.
Q. Is crypto-js npm compatible with TypeScript?
A. Yes, crypto-js npm is compatible with TypeScript. Type definitions are available, allowing for better integration and type checking in TypeScript projects.
Conclusion
Crypto-js npm has proven itself to be an invaluable tool for JavaScript developers seeking to implement cryptographic functions in their applications. Its versatility, extensive algorithm support, and cross-platform compatibility make it a popular choice for both browser and Node.js environments.
Throughout this guide, we’ve explored the core features of crypto-js npm, from basic hashing and encryption to more advanced use cases like digital signatures and secure communication channels. We’ve also discussed best practices, security considerations, and how crypto-js npm compares to other cryptographic libraries.
While it may not match the performance of native implementations for high-security or high-performance scenarios, crypto-js npm‘s ease of use and flexibility make it an excellent choice for a wide range of applications. As the cryptographic landscape continues to evolve, we can expect crypto-js npm to adapt and grow, maintaining its position as a go-to library for JavaScript cryptography.
By understanding the capabilities and limitations of crypto-js npm, developers can make informed decisions about its use in their projects, ensuring robust security while leveraging the power of JavaScript for cryptographic operations.
Disclaimer:
The information provided in this article on Crypto Wini is for educational purposes only. While we strive for accuracy, we cannot guarantee the completeness or reliability of the content. Always consult official documentation and security experts when implementing cryptographic solutions in your projects.
Also Read :
What Is Sui Crypto? A Comprehensive Guide To The Innovative Blockchain Platform
Binance Drops a Shocking BOMBSHELL: Say Goodbye to BUSD Forever
Breaking News: Binance Introduces MirrorX – The Future of VIP Trading in 2024
India’s Crypto Sting: How $10M in Digital Assets were Seized from E-Nugget Scam
Notcoin Mania: Telegram’s Viral Game Sparks $700M Crypto Frenzy
Binance’s Major Shake-Up: Delisting, Leverage Updates, and the Crypto Exodus
Banning Binance: India Blocks Foreign Exchanges, But What’s the Endgame?
India Crypto Regulation-Blocks Binance And 8 Other Crypto Exchanges: Know Why?
The Binance Settlement: You’ll Never Believe What Binance Is Hiding After Record Settlement